Introduction to REST API with Nodejs: A Beginner’s Tutorial
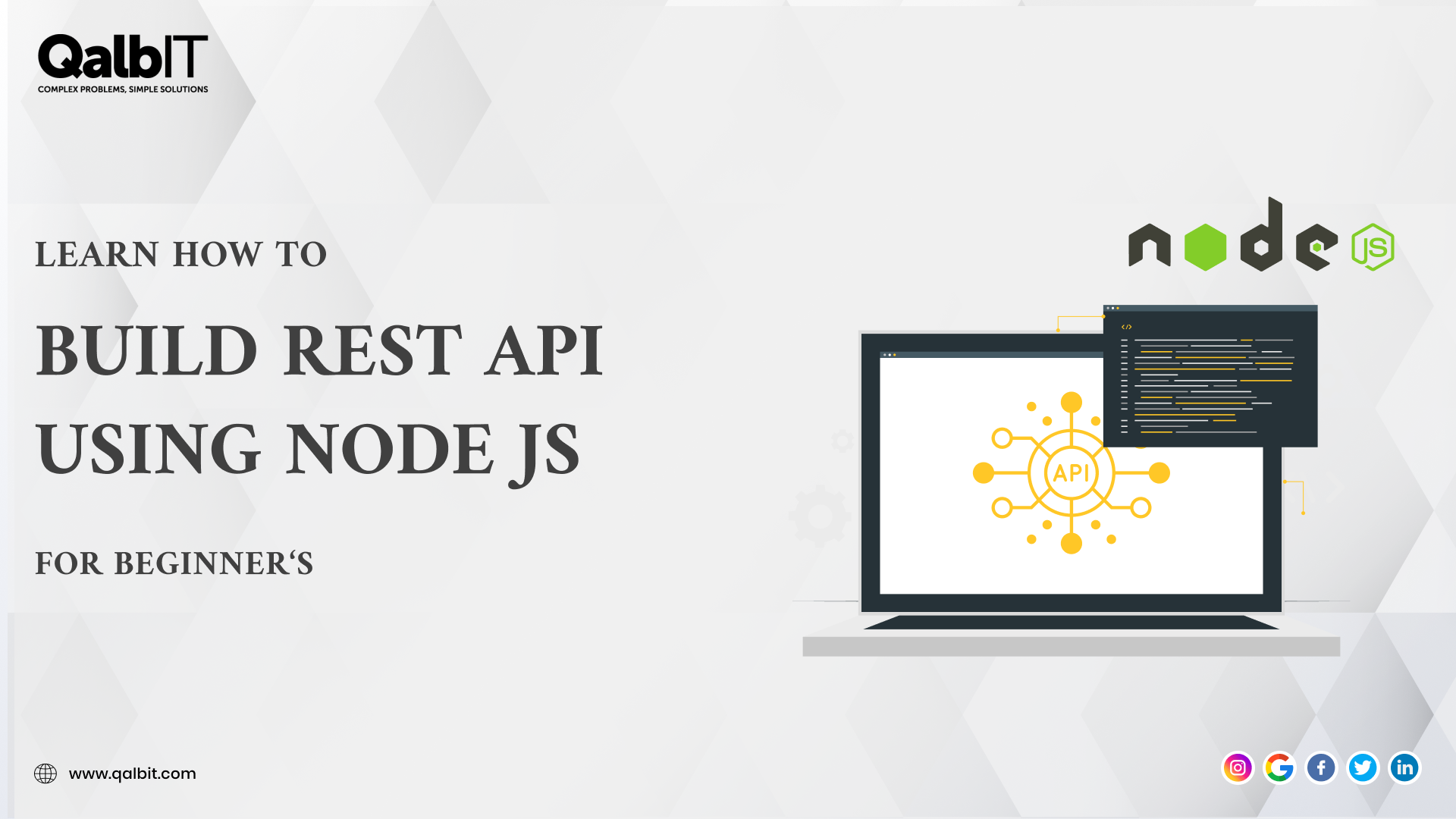
In this introductory lesson, we’ll walk through the methods of developing a Nodejs REST API. With building up the development environment to defining API endpoints, establishing a database connection, testing the API, and ultimately deploying it, we will go through the full procedure step-by-step.
I. Introduction
Before getting into the specifics, let’s quickly go over what a REST API is and its benefits. Web services are developed using the architectural design known as REST. It is a well-known method for creating web applications because it enables many systems to connect with one another and exchange data in a uniform manner.
It is a popular approach to building web services because it allows different systems to communicate with each other and share data in a standardized way
A well-liked platform for creating scalable web apps is Nodejs. It employs JavaScript, a language that many web developers are acquainted with, and it offers a variety of tools and modules that make it simple to construct online services. Nodejs is a fantastic option for creating REST APIs since it is compact, quick, and simple to use.
II. Establishing the development environment,
Setting up your development environment is the first step in developing a Nodejs REST API. Nodejs and npm must be installed, a new Nodejs project must be created, and the necessary dependencies must be installed. The steps are as follows:
1. Installing Nodejs with npm requires downloading Nodejs from its official website. Npm will also be accessible after installation. Run these commands in your terminal to see whether Nodejs and npm are set up:
node -v
npm -v
2. New Nodejs project creation: Create a new directory for your project in your terminal, then go there. To start a new Nodejs project, use the following command:
npm init
A package.json file will be generated and placed in your project directory.
3. Installing necessary dependencies The express package, which offers a straightforward and user-friendly API for developing online applications, is what we will need to employ in order to establish a REST API using Nodejs. Run the following command to install express:
npm install express
III. Creating the API endpoints
You may begin creating your API endpoints after your development environment has been configured. Clients utilize the endpoints, which are URLs, to communicate with your API. You may, for instance, have an endpoint that provides a list of all the items in your database or one that enables users to register for a new account.
You may build your endpoints using the Express framework. A selection of ways for specifying routes and managing HTTP requests are offered by Express. An example of a fundamental route handler that replies to a GET request is shown here:
app.get('/api/products', (req, res) => {
res.send('Here are all the products');
});
The /api/products endpoint is defined as a route in this example, and a function is provided to process the request. The res object is used to deliver a response back to the client, whereas the req object holds information about the request (such as the headers and query parameters).
In a manner similar to this, handlers may be defined for additional HTTP methods, such as POST, PUT, PATCH, and DELETE. As an example, consider the following when defining a handler for a POST request that adds a new user:
app.post('/api/users', (req, res) => {
// TODO: create a new user in the database
res.send('User created successfully');
});
To further increase the adaptability of your endpoints, you can also employ query strings and route parameters. Although query strings enable customers to transmit extra data as key-value pairs, route parameters allow you to define changeable sections of a URL. An example of how to create an endpoint that fetches a single product by ID is shown below:
app.get('/api/products/:id', (req, res) => {
const productId = req.params.id;
// TODO: retrieve product from the database using the ID
res.send(`Here is the product with ID ${productId}`);
});
This endpoint accepts an argument with the name ‘id’, as seen by the syntax ‘:id’. Using ‘req.params.id’, we can get the value of this field.
Express’s powerful middleware functionality lets you create functions that can manage requests before they get to your route handlers. Middleware may be used to manage problems, check user input, or authenticate users. Below is an example of a middleware procedure that determines if the client is allowed access to a certain endpoint:
function checkAuthorization(req, res, next) {
const apiKey = req.headers['x-api-key'];
if (apiKey !== 'secret') {
res.status(401).send('Unauthorized');
} else {
next();
}
}
app.get('/api/secure', checkAuthorization, (req, res) => {
res.send('You are authorized to access this endpoint');
});
In this example, we construct a middleware function named checkAuthorization that verifies the client’s x-API-key header for the presence of a valid API key. We execute the following method to forward the request to the following middleware or route handler if the key is valid. We provide the client with a 401 Unauthorized response code if the key is invalid.
IV. Connecting to a database
The majority of online applications need databases, and Nodejs offers a variety of modules that make working with databases simple. MongoDB, MySQL, a NoSQL database that stores data in a JSON-like format, is one of the most well-known databases.
You must register for a MongoDB Atlas account in order to begin using the cloud-based database service. You may establish a new cluster and set up your database settings after creating an account.
You must connect your database to your Nodejs application once you have configured it. Installing the mongoose module, which offers a quick and stylish method to deal with MongoDB from Nodejs, will enable you to achieve this.
Here’s an example of how to use mongoose to connect to a MongoDB database:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => {
console.log('Connected to database');
})
.catch((error) => {
console.error('Error connecting to database', error);
});
After you’ve established a connection to your database, you can use mongoose to communicate with it. You may use models to create, read, update, and remove data from your database and establish schemas to specify the data’s structure.
V. Testing the API
An overview of testing and its importance of it.
The process of developing software must include testing. By doing this, you can make sure that your application performs as intended and that any updates or added features don’t lead to unanticipated issues. Since it will be utilized by other apps or services that rely on it, testing your API is very crucial. Incorrect API operation may cause issues further down the line.
building a framework for testing (e.g. Mocha, Chai)
Many testing frameworks are available for Nodejs, however, in this part we’ll concentrate on Mocha and Chai. Chai is an assertion library that offers a collection of methods to make your tests more legible, whereas Mocha is a well-known testing framework that offers a straightforward and versatile interface for testing your code.
You may install Mocha and Chai using npm to get started with them:
npm install mocha chai --save-dev
Both packages will be installed as a result and their addition to your ‘package.json’ file’s and ‘devDependencies’ section. Next, you may add a test file to a new test directory you’ve created in your project for each endpoint you wish to test.
creating unit tests for every endpoint of an API
You should concentrate on individually evaluating each endpoint’s functionality while creating API tests. This will make it easier to guarantee that your tests are focused and particular and that any problems you discover may be more quickly identified and fixed.
You must send queries to your API using a test client in order to test your API endpoints. You may build a test client that can send queries to your API and check the answers using the ‘supertest’ module.
An example of a test for a GET call to the /users endpoint is shown below:
const request = require('supertest');
const app = require('../app');
describe('GET /users', () => {
it('responds with json', (done) => {
request(app)
.get('/users')
.set('Accept', 'application/json')
.expect('Content-Type', /json/)
.expect(200, done);
});
});
This test will issue a ‘GET’ call to the ‘/users’ endpoint and check if a JSON object with a status code of 200 is returned in the response.
Doing the tests and resolving any problems that surface
You may use the ‘npm test’ command to run your tests, which will run each test in your ‘test’ directory. If any tests fail, you should look into the reason and update your code as required to resolve it.
As testing is an iterative process, you may need to run your tests more than once to make sure your API functions as intended. In order to make sure that your API is reliable and capable of handling unforeseen data, it’s a good idea to build tests for edge situations and test it with various sorts of input.
VI. Deploying the API
- It’s time to launch your API once you’ve built it and tested it so that others may use it. Deploying your API entails opening it out to the public so that others may use and access it.
- You have a variety of choices for deploying an API, including hosting it on your own server or using cloud-based services like Heroku or Amazon. Since it is simple to use and offers a free tier for small projects, Heroku is a popular option for Nodejs apps.
- You must first register a Heroku account and install the Heroku CLI on your PC in order to deploy your API to Heroku. After that, you may develop a new app and use Git to publish your code to Heroku. Heroku will automatically create a container and execute your code inside of it. The container can then be accessible through a URL.
- Using Amazon is an additional alternative for hosting your API. Elastic Beanstalk and EC2 instances are only a few of the many services that Amazon provides for installing and executing applications. Amazon offers more flexibility and scalability for bigger applications, but it might be more difficult to set up and operate than Heroku.
- Make sure that your code is prepared for production before launching your API. This entails performance-enhancing your code, safeguarding your application with appropriate authentication and access rules, and setting up your environment.
VII. Concluding
Building robust, scalable apps that can be utilized by other developers and services may be accomplished by using Nodejs to create a REST API. You should now be familiar with the fundamentals of how to set up a development environment, establish API endpoints, connect to a database, test your API, and deploy it to a production environment after following the instructions in this book.
When it comes to Nodejs and REST API, there is always more to discover, therefore I urge you to keep investigating and playing with various frameworks, modules, and tools.
Want to create your Web application, Let’s Connect
https://qalbit.com/contact-us/
Frequently asked questions
Some best practices for designing a REST API with Node.js include keeping the API simple and easy to use, using descriptive and consistent endpoint names, implementing proper error handling and validation, and following the REST architectural style. It's also important to use good documentation and versioning to ensure that the API is maintainable and up-to-date. Additionally, it's recommended to use security measures such as authentication and authorization to protect the API from unauthorized access.
Yes, Node.js can be used to build a GraphQL API. In fact, some developers prefer to use GraphQL over REST due to its flexibility and efficiency in handling complex data queries. However, building a GraphQL API requires a different approach compared to a REST API, and it requires a different set of tools and libraries.
Some common performance issues to watch out for when building a Node.js REST API include inefficient database queries, excessive logging or debugging, and using synchronous functions where asynchronous functions would be more appropriate. Additionally, not caching frequently accessed data, not optimizing for memory usage, and not implementing load balancing or horizontal scaling can also lead to performance issues.