How to Quicken ReactJS Development Process and Increase Productivity
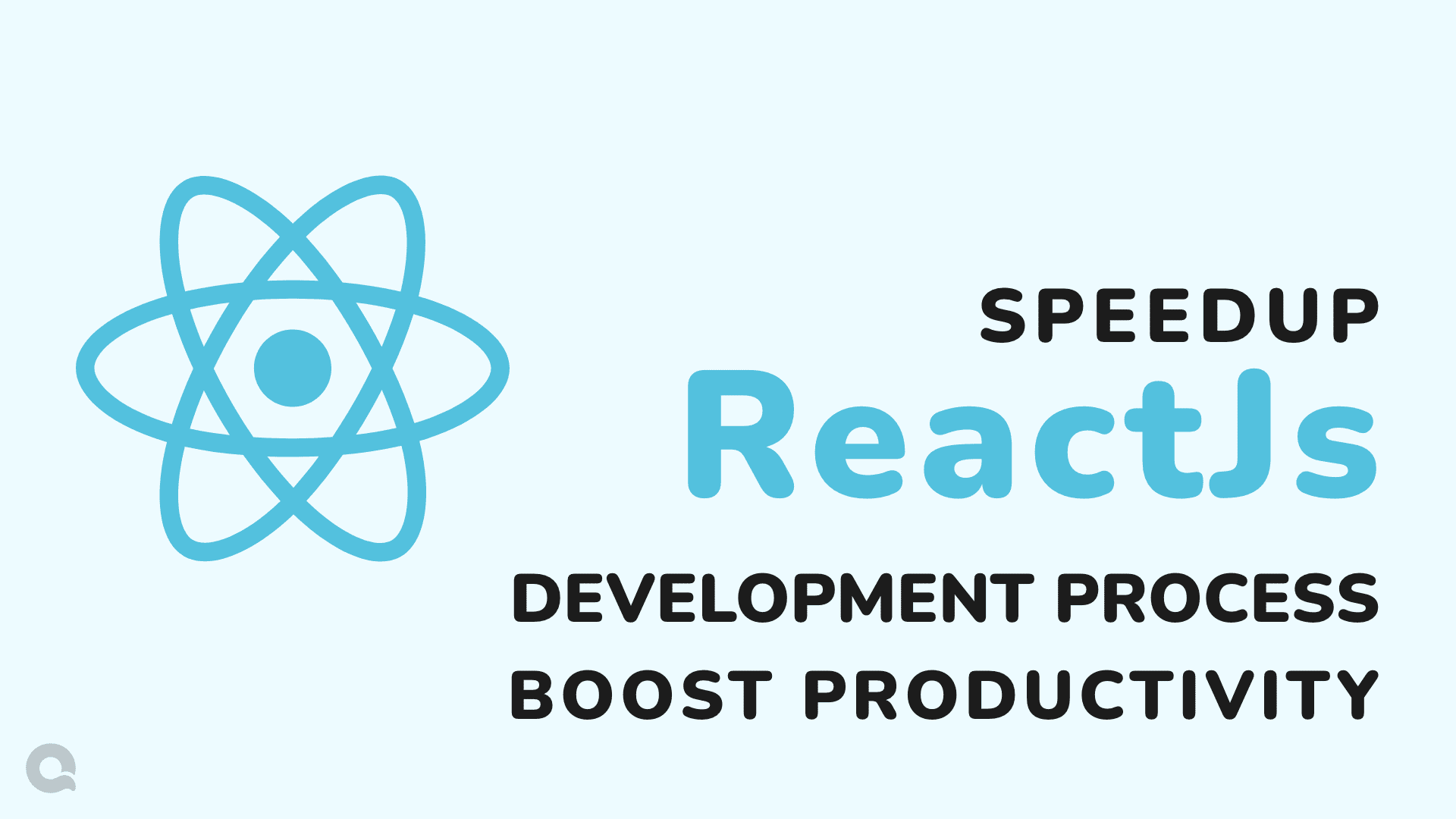
React uses several techniques that guarantee the minimization of the number of costly DOM operations to update the UI. That said, it ensures a faster user interface without specifically optimizing performance. Additional methods support the goal of speeding up your React application. Explore more regarding the reactjs development process and react js best practices in this blog.
A Highlight on some of the ReactJS Best Practices
1. Using Immutable Data Structures
Data immutability in the react js development process does not serve as an architecture or design pattern. Instead, it behaves as an opinionated way of writing code. The reactjs development strategy forces you to think about how you structure application data flow.
Data immutability revolves around a strict unidirectional data flow. Data immutability, from the functional programming world, applies to the design of front-end apps and has many benefits, such as:
- Zero side-effects;
- Immutable data objects become simpler to create, test, and use;
- prevention of the temporal coupling;
In the react js development process, there is significant use of the notion of component for maintaining the internal state of components. React builds and maintains representation of the rendered UI (Virtual DOM). At a stage after the component’s props alter, react takes into consideration comparing the fresh returned element with the earlier rendered piece. As soon as it gets the result that the two are not equal, React updates the DOM.
Consider a User List Component:
state = {
users: []
}
addNewUser = () =>{
/**
* Of course, not the correct way to insert
* new user in user list
*/
const users = this.state.users;
users.push({
userName: "robin",
email: "email@email.com"
});
this.setState({users: users});
}
The apprehension here is the pushing of a fresh user base onto the adjustable user base, serving as orientation to this.state.users. At every circumstance it must be noted to keep the React state immutable.
shouldComponentUpdate(nextProps, nextState) {
if (this.state.users !== nextState.users) {
return true;
}
return false;
}
Libraries you can use in such a stage include the Immutability Helper, Immutable.js, Seamless-immutable, React-copy-write.
2. Use of Multiple Chunk Files
For single-page React web apps, usually developers choose to bundle all front-end JavaScript code in a single minified file. This strategy is a perfect one for small to moderately sized web apps. However, it is worth noting that as the app starts to grow, delivering bundled JavaScript files to the browser becomes a time-consuming process.
When you are relying on using Webpack to build your React app, leverage its code-splitting capabilities that will serve the objective of separating your built app code into multiple “chunks.” After that, you can consider delivering them to the browser. Two types of splitting used include resource splitting and on-demand code splitting.
Resource splitting lets you split resource content into multiple files. A notable feature of Webpack is on-demand code splitting. Split code into a chunk of that. With that, there is a possibility to keep the initial download small, reducing the time taken to load the app.
3. Enabling Gzip on Web Server
Enabling Gzip on Web Server is a pro react js development strategy. React app bundles JS files that turn out to be very big to make the web page load faster. Enable Gzip on the web server.
Modern browsers automatically negotiate Gzip compression for HTTP requests. Gzip compression reduces the size of the transferred response, significantly reducing the time to download the resource. In addition to that, there is a possibility to reduce data usage for the client.
4. Using Production Mode Flag in Webpack
When you are using webpack 4, consider tweaking the mode option to production, referring to using the built-in optimization:
module.exports = {
mode: 'production'
};
Pass it as a CLI argument as the alternative attempt.
webpack --mode=production
This limits optimizations in react js web development, including the minification or removing development-only code, to libraries. This is an excellent method to not expose source code, file paths.
5. Using Dependency Optimization
Dependency optimization is well known as the top react js development strategy. When optimizing the application bundle size, check how much code you are utilizing from dependencies.
Moment.js includes localized files for multi-language support. In case you don’t need the support for the multiple languages, consider using the moment-locales-webpack-plugin to remove unused locales. Use lodash-webpack-plugin to remove unused functions.
Use React. Suspense and React.Lazy that will be favorable for Lazy Loading Components. Lazy loading serves as a great technique for optimizing and speeding up the render time. Lazy loading loads a component only when it is needed.
React bundled with the React.lazy API can render a dynamic import. React.lazy considers taking a function that must call a dynamic import().In addition to that, it will return a Promise resolving to a module with a default export.
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<div>
<Suspense fallback={<div>Loading....</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
6. React.memo for Component Memoization
React.memo serves as a great way of optimizing performance to help functional cache components. Computing strategies suggest that memoization is an optimization technique primarily to speed up computer programs with the storage of the results of expensive function calls and returning the cached result.
A function rendered using this technique saves the result in memory.functions are the functional components, while the arguments serve as props.
const MyComponent = React.memo(props => {
/* render only if the props changed */
});
7. Virtualization of a Large List Using react-window
When you’re choosing to render an enormous list of data, it slows down your app’s performance. Virtualization supports the objective here with the help of a library like react-window. React-window keeps on rendering only the items that are currently visible.
import React from 'react';
import { FixedSizeList as List } from 'react-window';
import './style.css';
const Row = ({ index, style }) => (
<div className={index % 2 ? 'ListItemOdd' : 'ListItemEven'} style={style}>
Row {index}
</div>
);
const Example = () => (
<List
className="List"
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{Row}
</List>
);
8. Detecting unnecessary rendering of components
Detection of the unnecessary rendering of components by using the “Why did you update” library is possible. When you are still not satisfied with the output of React performance tools, use the “why-did-you-update” library.
The purpose here is to hook into React and detect potentially unnecessary component renders. The library is an active stage and will start working on the console anytime a piece of state makes a component update.
9. Implementing shouldComponentUpdate for prevention of the unnecessary renders
Most performance-related issues find a permanent solution with the implementation of the smaller changes. These changes make your app faster. Bring in the performance improvement to your React app with the implementation of the shouldComponentUpdate method. shouldComponentUpdate serves as the react lifecycle hook to instruct React to avoid re-rendering a component.
There are many components with a complicated nested structure, and one item in the list keeps changing. Maintaining code with shouldComponentUpdate is tough at times as it turns out to be the major source of bugs.
10. Caching functions
You can go with calling the Functions in the React component JSX in the render method.
function expensiveFunc(input) {
...
return output
}
class ReactCompo extends Component {
render() {
return (
<div>
{expensiveFunc}
</div>
)
}
}
When the functions get expensive and take longer to execute, it will hang the rest of the re-render code to put an end to hampering the user’s experience.
The expensiveFunc gets rendered in the JSX. for each, the system re-render the function and renders the returned value on the DOM. The function is CPU-intensive. It’s worth noting that it will be called on every re-render, and React will have to wait to complete before running the rest of the re-rendering algorithm.
Cache the function’s input against the output. With this, it becomes easier to foster the continuous execution of the function as the same inputs occur again.
function expensiveFunc(input) {
...
return output
}
const memoizedExpensiveFunc = memoize(expensiveFunc)
class ReactCompo extends Component {
render() {
return (
<div>
{memoizedExpensiveFunc}
</div>
)
}
}
11. Using reselect selectors
Redux Js or reselect is the part of the reselect selectors. Getters in NuclearJS is the inspiration behind the Simple “selector” library for Redux (and others). Reselect optimizes Redux state management. Redux practices immutability. That said, it guarantees the creation of the new object references every time on action dispatch.
At times, it hampers performance because re-render will be triggered on components. The point you need to note here is that the Reselect library encapsulates the Redux state and tells React when to render and when to not.
Key Takeaways
Make your React web apps fast with the utilization of these strategies. There is a utilization of the leverage tools and techniques. React web app’s performance is also measurable with the simplicity of its components.
Before optimization of your app, understand how React components work. The react js best practices ensures the prevention of your component from re-rendering unnecessarily.
Frequently asked questions
You can migrate the website to ReactJS in three steps:
Step 1: Create a React Web App.
Step 2: Integrate Your Existing Web Pages.
Step 3: Verify Static Routes.
The rate is varied based on the country. In the US, the Yearly Average is $10000- $125000 while the Hourly average rate is $50-$60. In India the Yearly Average is $40000-$50000 while the Hourly average rate is $15-$25.
Future maintenance with ReactJS is fairly easy. Follow the best practices, including smart-dumb components. Though there are hassles of writing a lot of boilerplate, as a developer, you can rest assured that these codes make it easy to maintain in a large codebase, even with the presence of the collaborators.